- Android Background Restriction Is On
- Device Restriction Android
- Turn Off Background Restriction Android
Nougat (7.0) For Android 7.0 (Nougat), there is no way to set background data on or off of all apps at once. It has to be set for each individual app. From the Home screen, tap the app slider, then open “ Settings “. 'Background Restriction' (or 'Allow Background Activity' on some devices) is intended to stop ALL background activity regardless of whether your service has called setForeground There is no way around this setting. You cannot programmatically disable it. In case of OnePlus devices, you can see separate settings that restrict background services. To turn off restricting background services in Advanced, please refer to the following steps. Open the Settings on your OnePlus device and tap Advanced.
Whenever an app runs in the background, it consumes some of the device'slimited resources, like RAM. This can result in an impaired user experience,especially if the user is using a resource-intensive app, such as playing agame or watching video. To improve the user experience, Android 8.0(API level 26)imposes limitations on what apps can do while running in the background. Thisdocument describes the changes to the operating system, and how you can updateyour app to work well under the new limitations.
Overview
Many Android apps and services can be running simultaneously. For example, auser could be playing a game in one window while browsing the web in anotherwindow, and using a third app to play music. The more apps are running at once,the more load is placed on the system. If additional apps or services arerunning in the background, this places additional loads on the system, whichcould result in a poor user experience; for example, the music app might besuddenly shut down.
To lower the chance of these problems, Android 8.0 placeslimitations on what apps can do while users aren't directly interacting withthem. Apps are restricted in two ways:
Background Service Limitations: While an app is idle, there arelimits to its use of background services. This does not apply to foregroundservices, which are more noticeable to the user.
Broadcast Limitations: With limited exceptions, apps cannotuse their manifest to register for implicit broadcasts. They can stillregister for these broadcasts at runtime, and they can use the manifest toregister for explicit broadcasts targeted specifically at their app.
In most cases, apps can work around these limitations by using JobScheduler
jobs. This approach lets an app arrange toperform work when the app isn't actively running, but still gives the systemthe leeway to schedule these jobs in a way that doesn't affect the userexperience. Android 8.0 offers several improvements to JobScheduler
that make it easier to replace services andbroadcast receivers with scheduled jobs; for more information, seeJobScheduler improvements.
Background Service Limitations
Services running in the background can consume device resources, potentiallyresulting in a worse user experience. To mitigate this problem, the systemapplies a number of limitations on services.
The system distinguishes between foreground and background apps.(The definition of background for purposes of service limitations is distinctfrom the definition used by memorymanagement; an app might be in thebackground as pertains to memory management, but in the foreground as pertainsto its ability to launch services.)An app is considered to be in the foreground if any of the following is true:
- It has a visible activity, whether the activity is started or paused.
- It has a foreground service.
- Another foreground app is connected to the app, either by binding to one ofits services or by making use of one of its content providers. For example,the app is in the foreground if another app binds to its:
- Wallpaper service
- Notification listener
- Voice or text service
If none of those conditions is true, the app is considered to be in thebackground.
Note: These rules don't affectbound services in any way. Ifyour app defines a bound service, other components can bind to that servicewhether or not your app is in the foreground.
While an app is in the foreground, it can create and run both foreground andbackground services freely. When an app goes into the background, it has awindow of several minutes in which it is still allowed to create and useservices. At the end of that window, the app is considered to be idle. Atthis time, the system stops the app's background services, just as if the apphad called the services' Service.stopSelf()
methods.
Under certain circumstances, a background app is placed on a temporaryallowlist for several minutes. While an app is on the allowlist, it can launchservices without limitation, and its background services are permitted to run.An app is placed on the allowlist when it handles a task that's visible to theuser, such as:
- Handling a high-priorityFirebase Cloud Messaging (FCM)message.
- Receiving a broadcast, such as an SMS/MMS message.
- Executing a
PendingIntent
from a notification. - Starting a
VpnService
before the VPN app promotes itselfto the foreground.
Note:IntentService
is a service, and is therefore subject tothe new restrictions on background services. As a result, many apps that rely onIntentService
do not work properly when targeting Android8.0 or higher. For thisreason, AndroidSupport Library 26.0.0 introduces a newJobIntentService
class, which provides thesame functionality as IntentService
but uses jobs instead ofservices when running on Android 8.0 or higher.
In many cases, your app can replace background services with JobScheduler
jobs. For example, CoolPhotoApp needs to checkwhether the user has received shared photos from friends, even if the app isn'trunning in the foreground. Previously, the app used a background service whichchecked with the app's cloud storage. To migrate to Android 8.0 (API level 26),the developer replaces the background service with a scheduled job, which islaunched periodically, queries the server, then quits.
Prior to Android 8.0, the usual way to create a foreground servicewas to create a background service, then promote that service to the foreground.With Android 8.0, there is a complication; the system doesn't allow a backgroundapp to create a background service. For this reason, Android 8.0 introducesthe new method startForegroundService()
to start a new service inthe foreground. After the system has createdthe service, the app has five seconds to call the service's startForeground()
method to show the newservice's user-visible notification. If the app does not call startForeground()
within thetime limit, the system stops the service and declares the app to beANR.
Broadcast Limitations
If an app registers to receive broadcasts, the app's receiver consumesresources every time the broadcast is sent. This can cause problems if too manyapps register to receive broadcasts based on system events; a system event thattriggers a broadcast can cause all of those apps to consume resources in rapidsuccession, impairing the user experience. To mitigate this problem, Android7.0 (API level 24) placed limitations on broadcasts, as described inBackground Optimization.Android 8.0 (API level 26) makes these limitations more stringent.
- Apps that target Android 8.0 or higher can no longer registerbroadcast receivers forimplicit broadcasts in their manifest. An implicit broadcast is a broadcastthat does not target that app specifically. For example,
ACTION_PACKAGE_REPLACED
is an implicit broadcast,since it is sent to all registered listeners, letting them know that somepackage on the device was replaced. However,ACTION_MY_PACKAGE_REPLACED
is not an implicitbroadcast, since it is sent only to the app whose package was replaced, nomatter how many other apps have registered listeners for that broadcast. - Apps can continue to register for explicit broadcasts in their manifests.
- Apps can use
Context.registerReceiver()
at runtime to register a receiver for anybroadcast, whether implicit or explicit. - Broadcasts that require asignature permissionare exempted from this restriction, since these broadcasts are only sent toapps that are signed with the same certificate, not to all the apps on thedevice.
In many cases, apps that previously registered for an implicit broadcast canget similar functionality by using a JobScheduler
job.For example, a social photo app might need to perform cleanup on its data fromtime to time, and prefer to do this when the device is connected to a charger.Previously, the app registered a receiver for ACTION_POWER_CONNECTED
in its manifest; when the appreceived that broadcast, it would check whether cleanup was necessary. Tomigrate to Android 8.0 or higher, the app removes that receiver from itsmanifest. Instead, the app schedules a cleanup job that runs when the device isidle and charging.
Note: A number of implicit broadcasts are currently exempted from thislimitation. Apps can continue to register receivers for these broadcasts intheir manifests, no matter what API level the apps are targeting. For a list ofthe exempted broadcasts, see Implicit BroadcastExceptions.
Migration Guide
By default, these changes only affect apps that target Android 8.0 (API level26) or higher. However, users canenable these restrictions for any app from the Settings screen, even ifthe app targets an API level lower than 26. You may need to update your app tocomply with the new limitations.
Check to see how your app uses services. If your app relies on services thatrun in the background while your app is idle, you will need to replace them.Possible solutions include:
- If your app needs to create a foreground service while the app is inthe background, use the
startForegroundService()
method instead ofstartService()
. - If the service is noticeable by the user, make it a foreground service. Forexample, a service that plays audio should always be a foreground service.Create the service using the
startForegroundService()
method instead ofstartService()
. - Find a way to duplicate the service's functionality with a scheduled job. Ifthe service is not doing something immediately noticeable to the user, youshould generally be able to use a scheduled job instead.
- Use FCM to selectivelywake your application up when network events occur, rather than polling in thebackground.
- Defer background work until the application is naturally in the foreground.
Review the broadcast receivers defined in your app's manifest. If your manifestdeclares a receiver for an implicit broadcast, you must replace it. Possiblesolutions include:
- Create the receiver at runtime by calling
Context.registerReceiver()
, insteadof declaring the receiver in the manifest. - Use a scheduled job to check for the condition that would have triggered theimplicit broadcast.
By default several apps running on the background even we don’t use it. Want to disable the background data restriction app? Here’s how to turn off background running apps on the latest android devices. This apps running in the background is consuming battery and slow down your phone speed also.
By default enable usage of mobile data in the background in your android device. You can turn off background apps activity on android Pie 9.0 using apps and notification settings. Also, allow unrestricted data access when data saver in turn on in your phone. Follow the below-given step by step process to disable apps background data Android 9 Pie.
Read Also:
How to Restrict Apps Background Data on Android 9.0 Pie
You can stop android apps that running in the background & usage more data using below given settings.
Restrict background data for individual apps on Android 10
You can turn off apps background data android 10 using below-given apps & notification settings.
Step 1: Go to Settings.
Step 2: Tap on Apps & notifications.
Step 3: Tap on See all apps.
Step 4:Select the app from a list.
Step 5: Tap on Mobile data and Wi-Fi.

Step 6: Toggle off Background data.
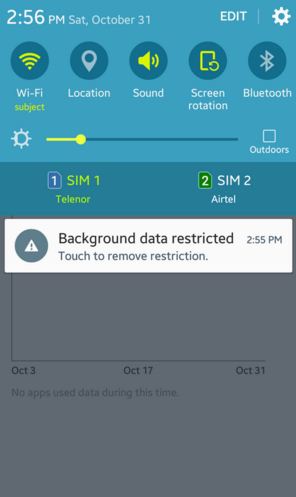
Clear all recently open apps that running in the background on Android 10
Step 1: Open recent apps in your Android device.
Step 2: Swipe screen right side until the Clear all show.
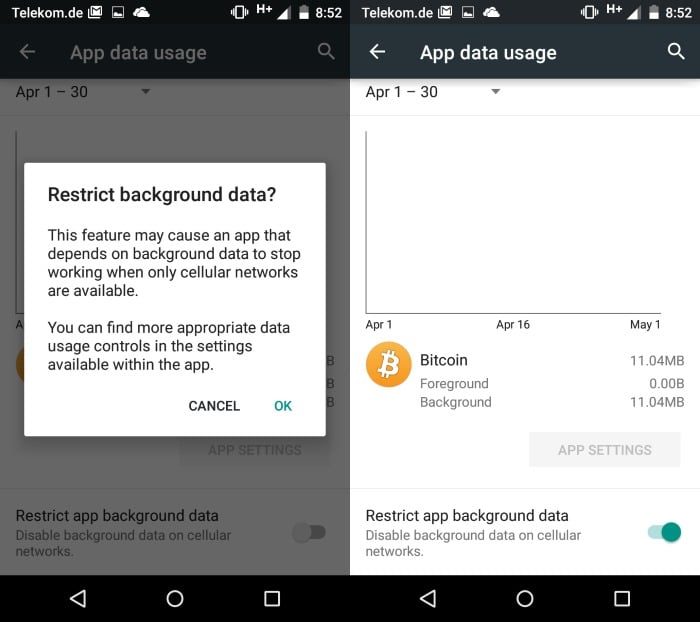
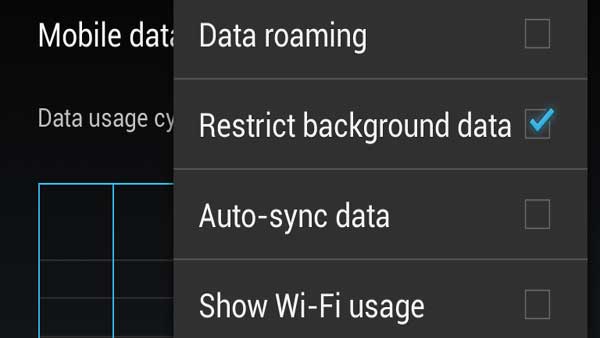
Step 3: Tap on Clear all to disable running background apps.
For Android 9 Pie:
In the latest Android 9 Pie devices, you can set an app time limit to limit app use in your device using the Digital Wellbeing feature. Check out below settings to turn off background data on android 9.0 Pie devices.
Step 1: Swipe down notification shade twice and tap the Settings gear icon.
Step 2: Tap Network & internet.
Step 3: Tap Data usage.
Here you can see individual app usage data and Wi-Fi data usage in your device.
Step 4: Tap App data usage under the mobile section.
Now appear all apps data usage in your android Pie 9.0 device. You’ll individually restrict apps background activity in your device.
Step 5: Tap app you want to stop apps running in the background.
By default enablebackground data in your android 9 Pie and all other devices.
Step 6: Turn off toggle “Background data” to background data restriction app.
You can also check Wi-Fi usage data using this setting. And also turn off Wi-Fi data usage by apps on your phone.
How to restrict Wi-Fi background data on Android 9 Pie
Android Background Restriction Is On
To restrict background data Wi-Fi on the android Pie 9.0 device, apply below-given settings.
Device Restriction Android
Settings > Network & internet > Data usage > Wi-Fi data usage > Tap app to disable background data Wi-Fi > Turn off toggle background data
Turn Off Background Restriction Android
And that’s all. I hope this tutorial useful to disable apps background data Android 9 Pie. If you have any questions, tell us in below comment box. Stay and connect with us for the latest updates.
